Overview
This is a simple sample to scrape all images from a website by Python. While “find_element” of Clicknium is for locating a unique UI control, “find_elements” can be used to locate multiple UI controls with one locator.
This example shows a basic usage scenario for this concept, and another blog shows advanced usage in capturing list of elements.
Practice Walkthrough
Stpe1: With the Clicknium recorder, record a locator for any image from the Clicknium homepage.
Stpe2: Change the locator to match any image of the web page. As the below picture showing, keeping the tag property only and dropping the others.
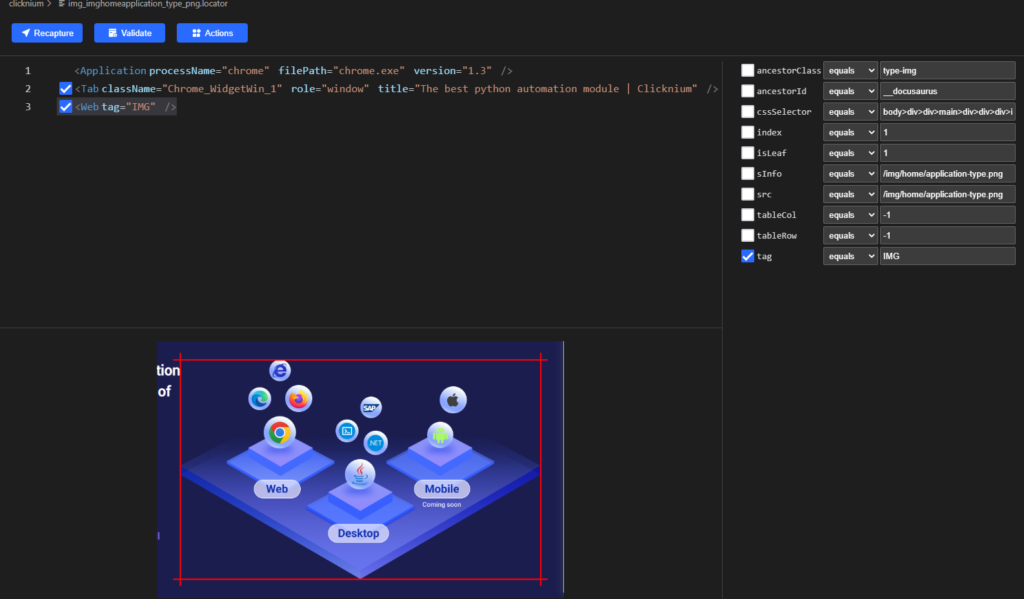
Stpe3: With the updated locator, we can write the code as below to get all image elements in the web page and download them all. from clicknium import clicknium, locator
import requests
# open web page
tab = clicknium.chrome.open("https://www.clicknium.com")
# get all images by the locator
imgs = tab.find_elements(locator.chrome.clicknium.img)
# get image url from its property 'src'
sources = []
for img in imgs:
source = img.get_property("src")
if source:
sources.append(source)
# download all images by the given src
for source in sources:
webs = requests.get(websiteUrl + source)
open('images/' + source.split('/')[-1], 'wb').write(webs.content)
More
This sample shows a basic use case for locating multiple elements. To capture some more complicated elements, you may refer this blog to know how to implement it by using Clicknium ‘Similar elements’ feature.